Page
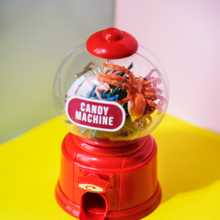
Naming things real good
Naming stuff: Object Oriented Basics
updated 6 months ago
Naming stuff: Object Oriented Basics
Let’s look at Object/class design by example…
The Situation
Have you ever designed a data model
(in code, Sql, or excel worksheets)?
Does the following look familiar?
*** anti-pattern - don't copy-paste ***
* User
- id
- avatarUrl
- name
- email
- password
* Agent
- id
- primaryPhoto
- name
- email
- agentEmail
- agentPhoneMain
- agentEmailPrimary
- agentPhonePrimary
- agentAddressFull
- agentCompanyName
- agentCompanyAddress
- *userEmail* - 'Pointer' to User table ^^^
Where’s the bug?
Well, technically there’s no bug, simply data in need of re-organization.
Does the following sound familiar?
- Any change to your app will necessitate hours of arduous debugging.
- Any changing requirements will result in:
Why is naming a field agentEmailPrimary
so bad?
For starters, you are not creating an entirely new thing unto the universe. Over-specificity has some traps:
-
‘Locked’ into highly specific name, means
agentEmailPrimary
probably make your views and related code 0% reusable, and featuring annoyingly recurring bugs like: -
Data not syncing between tables (not obvious if
user.email
needs to propagate toagent.agentEmail
or vice-versa - nevermind complexity of manually implementing where & how to enforce this ‘logic’ …) -
Validation rules/logic are likely duplicated & inconsitent.
-
Increasingly, your project will resemble a shaky Jenga tower.
-
Fragility piles up with every single new file, as an extremely high attention to detail is required for even trivial changes
-
agentEmailPrimary
could mean a few different things. Avoid ambiguity with shorter names. -
Watch out for silly excess wording.
Primary
? Just leads to more questions: Is there a Secondary? Is it for their Primary Next-of-kin?
Enough words Dan, what should it look like instead?
A Solution
// Consolidated Schema:
User
- id
- role: ['agent', 'lead', 'admin']
- name
- phone
- address
- email
- password
- company
- name
- address
I removed the Agent
table, as it didn’t contain fields which were unique to Agents. And the User.company
object (with .name
, .address
) emerged once the naming was cleaned up.
Some guiding principles:
- Eliminate unnecessary tables. Do you really need to have a
statuses
table? When you could add astatus::VARCHAR(8)
field on theUser
table? It’s ok, use the extra bytes per row. - Try merge related tables. Data
- Delete redundant data collection (e.g. remove
ActivityLogs
table if replaced by an Analytics solution.) - Try keeping all field names to a single word/noun/pro-noun. It’s ok to rely on the context provided by the table. (e.g.
PersonalAccount.email
vsBusinessAccount.email
- the context is provided by the table name.) - There is no such thing as
Agent.agentEmail
orAgent.agentPhonePrimary
. Period. Say it with me: “it’semail
&phone
.” - By using Highly Specific Names, you cast-in-stone a specific level of
code-reusability
anddurability
, well, specifically ZERO %. - You aren’t doing yourself any favors with crap like this
User.profileSummaryEmail
. 💞
Recommended reading includes: